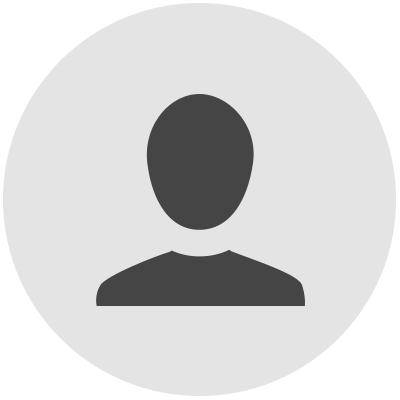
Just to add. Get the seconds since epoch (Jan 1 1970) for any given date (e.g Oct 21 1973). date -d"Oct 21 1973" +%s. Convert the number of seconds back to date. date --date @120024000. The command date is pretty versatile. Another cool thing you can do with date (shamelessly copied from date --help).
Share, comment, bookmark or report
466. To get the number of full minutes, divide the number of total seconds by 60 (60 seconds/minute): const minutes = Math.floor(time / 60); And to get the remaining seconds, multiply the full minutes with 60 and subtract from the total seconds: const seconds = time - minutes * 60; Now if you also want to get the full hours too, divide the ...
Share, comment, bookmark or report
There's no built-in formatter for timedelta objects, but it's pretty easy to do it yourself:. days, seconds = duration.days, duration.seconds hours = days * 24 + seconds // 3600 minutes = (seconds % 3600) // 60 seconds = seconds % 60
Share, comment, bookmark or report
You can convert seconds to days by dividing by 86400. You can convert seconds to hours by dividing by 3600, but you need to get the remainder (by subtracting off the total days converted to hours) You can convert seconds to minutes by dividing by 60, but you need to get the remainder (by subtracting off the total hours converted to minutes)
Share, comment, bookmark or report
I need to convert seconds to"Hour:Minute:Second". For example:"685" converted to"00:11:25" How can I achieve this?
Share, comment, bookmark or report
1 minute = 60 seconds. 1 hour = 3600 seconds (60 * 60) 1 day = 86400 second (24 * 3600) First divide the input by 86400. If you you can get a number greater than 0, this is the number of days. Again divide the remained number you get from the first calculation by 3600. This will give you the number of hours.
Share, comment, bookmark or report
If you place the number of seconds in a TimeSpan object, you can quite easily extract the days, hours, minutes, seconds and even fractional seconds directly using TimeSpan.toString() method. Using an identical form and object names, I used the following code to achieve the desired results.
Share, comment, bookmark or report
let startTime = new Date(timeStamp1); let endTime = new Date(timeStamp2); to get the difference between the dates in seconds ->. let timeDiffInSeconds = Math.floor((endTime - startTime) / 1000); but this porduces results in utc (for some reason that i dont know). So you have to take account for timezone offset, which you can do so by adding.
Share, comment, bookmark or report
I have a function that returns information in seconds, but I need to store that information in hours:minutes:seconds. Is there an easy way to convert the seconds to this format in Python?
Share, comment, bookmark or report
I use this in python to convert a float representing seconds to hours, minutes, seconds, and microseconds. It's reasonably elegant and is handy for converting to a datetime type via strptime to convert. It could also be easily extended to longer intervals (weeks, months, etc.) if needed.
Share, comment, bookmark or report
Comments